UI Framework for Rapid Layout Development
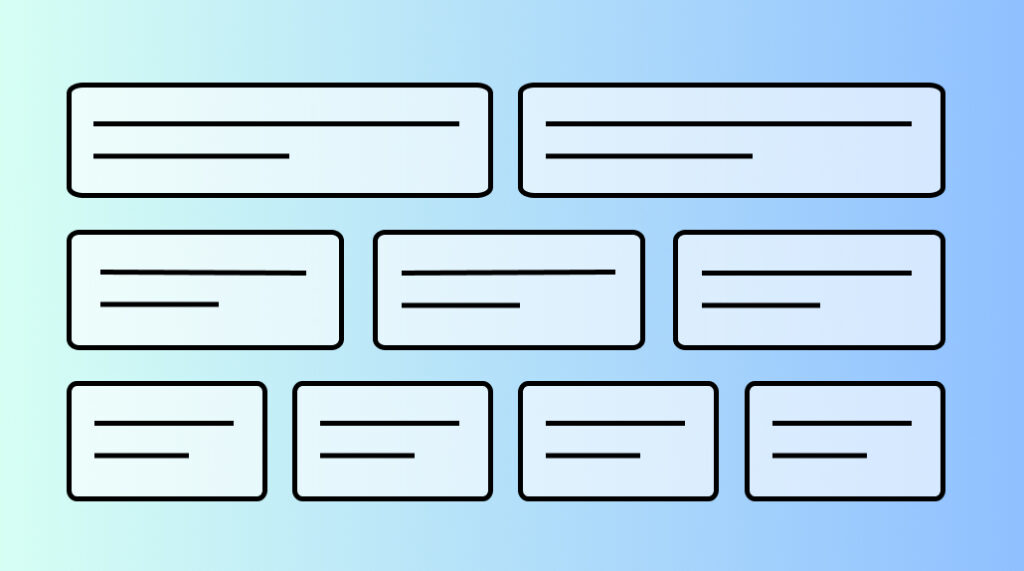
Modern web applications demand efficient UI development workflows that can scale. This UI framework provides a comprehensive set of CSS utilities designed to streamline interface development, from basic layouts to complex UI components. By combining a powerful grid system with extensive UI utilities, developers can rapidly build consistent, responsive user interfaces without writing custom CSS.
Key Benefits
- Rapid UI Development: Build interfaces faster with ready-to-use utility classes
- Consistent Design: Maintain visual consistency across your application
- Responsive by Default: Built-in responsive modifiers for all screen sizes
- Production-Ready: Optimized for real-world UI development
- Framework Agnostic: Works with any JavaScript framework or vanilla HTML
Features at a Glance
- Complete grid system with flexible layouts
- Comprehensive spacing and positioning utilities
- Typography and display helpers
- UI-focused helper classes for common interface patterns
- Responsive modifiers for all components
- Accessibility-friendly class structure
Responsive Breakpoints
The system uses three main breakpoints:Mobile: max-width: 40em (640px)
Tablet: 40.0625em to 64em (641px to 1024px)
Desktop: min-width: 64.0625em (1025px+)
Grid System
Basic Column Layouts
The system provides pre-built classes for common column layouts:
<div class="column-2">
<div>Column 1 content</div>
<div>Column 2 content</div>
</div>
Available column layouts include:
column-2
: Two equal columnscolumn-3
: Three equal columnscolumn-4
: Four equal columnscolumn-5
: Five equal columns
Advanced Grid Ratios
For more specific layout needs, the system includes ratio-based grid classes:
grid-3-9
: 25%/75% splitgrid-4-8
: 33.33%/66.66% splitgrid-5-7
: 41.66%/58.33% splitgrid-7-5
: 58.33%/41.66% splitgrid-8-4
: 66.66%/33.33% splitgrid-9-3
: 75%/25% split
CSS Grid Examples:
2 column content
This is an example of 2 column content.
Column 1
Example content of column 1. Here we have a paragraph about column 1.
Column 2
Example content of column 2. Here we have a paragraph about column 2.
3 column content
This is an example of 3 column content.
Column 1
Example content of column 1. Here we have a paragraph about column 1.
Column 2
Example content of column 2. Here we have a paragraph about column 2.
Column 3
Example content of column 3. Here we have a paragraph about column 3.
4 column content
This is an example of 4 column content.
Column 1
Example content of column 1. Here we have a paragraph about column 1.
Column 2
Example content of column 2. Here we have a paragraph about column 2.
Column 3
Example content of column 3. Here we have a paragraph about column 3.
Column 4
Example content of column 4. Here we have a paragraph about column 4.
5 column content
This is an example of 5 column content.
Column 1
Example content of column 1. Here we have a paragraph about column 1.
Column 2
Example content of column 2. Here we have a paragraph about column 2.
Column 3
Example content of column 3. Here we have a paragraph about column 3.
Column 4
Example content of column 4. Here we have a paragraph about column 4.
Column 5
Example content of column 5. Here we have a paragraph about column 5.
3 / 9 grid layout
This is an example of 3 / 9 grid layout.
Column 1
Example content of column 1. Here we have a paragraph about column 1.
Column 2
Example content of column 2. Here we have a paragraph about column 2.
4 / 8 grid layout
This is an example of 4 / 8 grid layout.
Column 1
Example content of column 1. Here we have a paragraph about column 1.
Column 2
Example content of column 2. Here we have a paragraph about column 2.
5 / 7 grid layout
This is an example of 5 / 7 grid layout.
Column 1
Example content of column 1. Here we have a paragraph about column 1.
Column 2
Example content of column 2. Here we have a paragraph about column 2.
7 / 5 grid layout
This is an example of 7 / 5 grid layout.
Column 1
Example content of column 1. Here we have a paragraph about column 1.
Column 2
Example content of column 2. Here we have a paragraph about column 2.
8 / 4 grid layout
This is an example of 8 / 4 grid layout.
Column 1
Example content of column 1. Here we have a paragraph about column 1.
Column 2
Example content of column 2. Here we have a paragraph about column 2.
9 / 3 grid layout
This is an example of 9 / 3 grid layout.
Column 1
Example content of column 1. Here we have a paragraph about column 1.
Column 2
Example content of column 2. Here we have a paragraph about column 2.
Helper Classes
Spacing System
The system includes comprehensive spacing classes for margin and padding:
Column classes:
column-2 = 2 column layout
column-3 = 3 column layout
column-4 = 4 column layout
column-5 = 5 column layout
column-1-t = 1 column tablet
column-3-t = 3 column tablet
column-no-gap = no gap in columns
column-no-row-gap-m = no row gap on mobile
column-no-row-gap-t = no row gap on tablet
column-no-row-gap-t-down = no row gap on tablet down
column-row-gap-3-m = row gap of 3rem on mobile
column-row-gap-4-m = row gap of 4rem on mobile
column-row-gap-3-t = row gap of 3rem on tablet
column-row-gap-4-t = row gap of 4rem on tablet
column-row-gap-3-d-up = row gap of 3rem desktop up
column-row-gap-4-d-up = row gap of 4rem desktop up
column-span-2-d = grid-column: span 2; on desktop
column-span-3-d = grid-column: span 3; on desktop
column-span-4-d = grid-column: span 4; on desktop
Grid classes:
grid-2-10 = 2 / 10 grid layout
grid-3-9 = 3 / 9 grid layout
grid-4-8 = 4 / 8 grid layout
grid-5-7 = 5 / 7 grid layout
grid-7-5 = 7 / 5 grid layout
grid-8-4 = 8 / 4 grid layout
grid-9-3 = 9 / 3 grid layout
grid-10-2 = 10 / 2 grid layout
grid-8-t = 66.66% columns on tablet
grid-9-t = 75% columns on tablet
grid-2-10-t = 2 / 10 grid on tablet
grid-2-10-t-down 2 / 10 grid tablet down
grid-8-4-t = 8 / 4 grid on tablet
grid-row-1-m = make item show up first on mobile
grid-row-2-m = make item show up second on mobile
grid-row-1-t-down = make item show up first tablet down
grid-row-2-t-down = make item show up second tablet down
Padding classes (any number from 0 to 200):
p-10 = padding: 10px;
pt-10 = padding-top: 10px;
pb-10 = padding-bottom: 10px;
pl-10 = padding-left: 10px;
pr-10 = padding-right: 10px;
Margin classes (any number from 0 to 200):
m-10 = margin: 10px;
mt-10 = margin-top: 10px;
mb-10 = margin-bottom: 10px;
ml-10 = margin-left: 10px;
mr-10 = margin-right: 10px;
Padding classes – tablet (any number from 0 to 200):
p-10-t = padding: 10px;
pt-10-t = padding-top: 10px;
pb-10-t = padding-bottom: 10px;
pl-10-t = padding-left: 10px;
pr-10-t = padding-right: 10px;
Margin classes – tablet(any number from 0 to 200):
m-10-t = margin: 10px;
mt-10-t = margin-top: 10px;
mb-10-t = margin-bottom: 10px;
ml-10-t = margin-left: 10px;
mr-10-t = margin-right: 10px;
Padding classes – mobile (any number from 0 to 200):
p-10-m = padding: 10px;
pt-10-m = padding-top: 10px;
pb-10-m = padding-bottom: 10px;
pl-10-m = padding-left: 10px;
pr-10-m = padding-right: 10px;
Margin classes – mobile(any number from 0 to 200):
m-10-m = margin: 10px;
mt-10-m = margin-top: 10px;
mb-10-m = margin-bottom: 10px;
ml-10-m = margin-left: 10px;
mr-10-m = margin-right: 10px;
Margin negative classes (any number from 0 to 200):
mt-neg-10 = margin-top: -10px;
mb-neg-10 = margin-bottom: -10px;
ml-neg-10 = margin-left: -10px;
mr-neg-10 = margin-right: -10px;
Margin negative tablet and mobile (any number from 0 to 200):
mt-neg-10-t = margin-top: -10px; (tablet only)
mb-neg-10-t = margin-bottom: -10px; (tablet only)
ml-neg-10-t = margin-left: -10px; (tablet only)
mr-neg-10-t = margin-right: -10px; (tablet only)
mt-neg-10-m = margin-top: -10px; (mobile only)
mb-neg-10-m = margin-bottom: -10px; (mobile only)
ml-neg-10-m = margin-left: -10px; (mobile only)
mr-neg-10-m = margin-right: -10px; (mobile only)
Background color classes:
bg-color-grey = background-color: #EEEFEF;
bg-color-white = background-color: #ffffff;
Display classes
hide = display: none;
d-none = display: none;
d-none-m = display none on mobile
d-none-t = display none on tablet
d-none-t-up = display none on tablet up
d-none-t-down = display none on tablet down
d-none-d-up = display none on desktop up
d-grid = display: grid;
d-block = display: block;
d-block-m = display block on mobile
d-block-t = display block on tablet
d-block-t-up = display block on tablet up
d-block-t-down = display block on tablet down
d-block-d-up = display block on desktop up
sr-only = screen readers only
Display classes continued and position classes
d-flex = display: flex;
d-flex-d = display: flex; (desktop only)
d-inline-block = display inline block
d-inline-block-m = display inline block on mobile
d-inline-block-t = display inline block on tablet
d-inline-block-t-up = display inline block on tablet up
d-inline-block-t-down = display inline block on tablet down
d-inline-block-d-up = display inline block on desktop up
p-relative = position: relative;
p-absolute = position: absolute;
z-index-2 = z-index: 2;
z-index-9999 = z-index: 9999;
overflow-hidden = overflow: hidden;
Float classes:
f-none = float: none;
f-l = float: left;
f-l-m = float: left; (mobile)
f-l-t = float: left; (tablet)
f-l-t-down = float: left; (tablet down)
f-l-d-up = float: left; (desktop up)
f-r = float: right;
f-r-t = float: right; (tablet)
f-r-m = float: right; (mobile)
f-r-t-down = float: right; (tablet down)
f-r-d-up = float: right; (desktop up)
Font title classes:
h1 = h1 level title
h2 = h2 level title
h3 = h3 level title
h4 = h4 level title
Border radius classes
brd-r-1 = border radius 1px
brd-r-2 = border radius 2px
brd-r-3 = border radius 3px
brd-r-4 = border radius 4px
brd-r-5 = border radius 5px
brd-r-6 = border radius 6px
brd-r-8 = border radius 8px
brd-r-10 = border radius 10px
brd-r-b-10 = border radius bottom 10px
Font weight classes:
fw-300 = font-weight: 300;
fw-400 = font-weight: 400;
fw-500 = font-weight: 500;
fw-600 = font-weight: 600;
fw-700 = font-weight: 700;
Other classes
container = sets a max width container for wrapping content
c-black = color: #000;
c-white = color: #fff;
c-white-m = color: #fff; (mobile)
box-shadow = box-shadow: 0px 1px 16px rgba(0, 0, 0, 0.15);
text-break = word-break: break-word;
underline-m = text-decoration: underline; (mobile)
w-100-p = width 100%
w-100-p-m = width 100% on mobile
h-100-p = height 100%
h-auto-m = height auto on mobile
t-center = text center
t-center-m = text center mobile
t-center-d-up = text center desktop
t-right = text right
t-right-m = text right mobile
t-right-d-up = text right desktop
tt-uppercase = text transform uppercase
ai-center = align items center
jc-center = justify content center
Best Practices
- Consistent Spacing: Use the spacing classes rather than custom margins/paddings to maintain consistency across your site.
- Responsive Design: Always consider mobile-first and use responsive modifiers appropriately:
<div class="column-3">
<!-- 3 columns on desktop, 2 column on tablet, 1 column on mobile -->
</div>
- Grid Gaps: Use the built-in gap controls:
<div class="column-2 column-no-gap">
<!-- 2 columns on desktop, 2 columns on tablet, 1 column on mobile, No gap between columns -->
</div>
- Accessibility: Use semantic HTML and take advantage of the
.sr-only
class for screen reader content.
Conclusion
This CSS grid and helper classes system provides a robust foundation for building modern websites. Its combination of flexible grid layouts, comprehensive spacing utilities, and responsive modifiers makes it a powerful tool for creating maintainable and scalable CSS architectures.
The system’s strength lies in its simplicity and consistency – by using these predefined classes, you can rapidly build layouts while maintaining a coherent design system across your project.
Remember to always consider mobile-first development and leverage the responsive modifiers to create truly adaptive layouts that work across all devices.
To see it in action, you can view the UI Framework for Rapid Layout Development example either on Codepen or GITHUB.